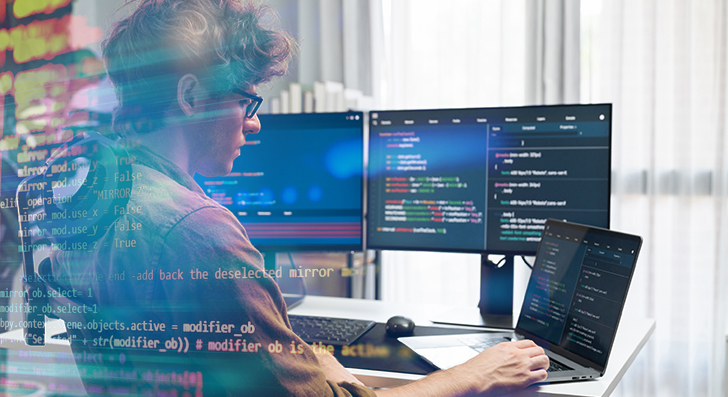
Scalability indicates your application can manage growth—extra people, a lot more information, and more targeted visitors—devoid of breaking. Like a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and sensible guideline that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability isn't anything you bolt on later—it ought to be part of your respective plan from the start. Many apps fail whenever they grow rapidly because the initial structure can’t manage the additional load. As being a developer, you'll want to think early about how your procedure will behave under pressure.
Start off by designing your architecture for being adaptable. Steer clear of monolithic codebases the place all the things is tightly connected. Alternatively, use modular design or microservices. These patterns split your application into lesser, independent areas. Each individual module or services can scale on its own devoid of influencing The entire technique.
Also, give thought to your database from day a single. Will it need to have to take care of one million users or perhaps 100? Choose the correct sort—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, even if you don’t want them nevertheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t publish code that only will work underneath latest disorders. Think about what would happen Should your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use structure styles that assist scaling, like concept queues or function-pushed devices. These support your application manage a lot more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you are not just preparing for success—you might be cutting down long run complications. A effectively-planned system is less complicated to take care of, adapt, and mature. It’s superior to get ready early than to rebuild later on.
Use the proper Databases
Deciding on the appropriate database is a vital Section of making scalable programs. Not all databases are constructed a similar, and utilizing the Incorrect you can sluggish you down or even induce failures as your app grows.
Commence by comprehending your details. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. They're robust with interactions, transactions, and consistency. In addition they help scaling techniques like read through replicas, indexing, and partitioning to handle additional visitors and details.
Should your information is a lot more flexible—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured facts and can scale horizontally far more easily.
Also, contemplate your browse and create designs. Are you carrying out numerous reads with much less writes? Use caching and read replicas. Are you presently handling a large produce load? Look into databases that will cope with high create throughput, as well as party-based knowledge storage units like Apache Kafka (for temporary information streams).
It’s also sensible to Assume in advance. You might not need Sophisticated scaling functions now, but picking a databases that supports them means you won’t require to switch later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your facts determined by your obtain styles. And normally keep track of database overall performance as you develop.
In brief, the proper database depends upon your app’s structure, velocity requires, and how you anticipate it to develop. Take time to select sensibly—it’ll help save many difficulties later on.
Optimize Code and Queries
Quick code is essential to scalability. As your app grows, every compact hold off adds up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s vital that you Develop efficient logic from the beginning.
Get started by producing clear, easy code. Avoid repeating logic and take away everything pointless. Don’t pick the most intricate Remedy if a straightforward just one will work. Maintain your capabilities limited, focused, and straightforward to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes as well extensive to operate or utilizes far too much memory.
Following, look at your databases queries. These often sluggish things down in excess of the code itself. Ensure that Every question only asks for the data you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly across big tables.
When you notice the identical details becoming requested time and again, use caching. Store the outcome briefly applying resources like Redis or Memcached and that means you don’t really have to repeat high-priced functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app much more productive.
Make sure to take a look at with significant datasets. Code and queries that operate high-quality with a hundred records may crash after they have to deal with 1 million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These measures support your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and a lot more targeted traffic. If anything goes by one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment aid keep your app fast, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. In place of one particular server undertaking each of the function, the load balancer routes users to different servers dependant on availability. What this means is no single server gets overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, here or cloud-centered alternatives from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it can be reused immediately. When end users request a similar data once more—like an item webpage or a profile—you don’t really need to fetch it with the database when. It is possible to serve it with the cache.
There are two popular varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
two. Consumer-side caching (like browser caching or CDN caching) outlets static files near the user.
Caching lowers databases load, enhances velocity, and tends to make your application more productive.
Use caching for things which don’t modify generally. And usually ensure that your cache is up to date when details does alter.
Briefly, load balancing and caching are easy but strong applications. With each other, they assist your application deal with far more buyers, stay rapidly, and Get better from problems. If you intend to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you require tools that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to purchase hardware or guess potential capability. When site visitors will increase, it is possible to insert additional methods with just a couple clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also give products and services like managed databases, storage, load balancing, and stability applications. You may center on constructing your app as opposed to handling infrastructure.
Containers are another key Software. A container deals your application and almost everything it has to run—code, libraries, configurations—into just one unit. This makes it quick to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs a number of containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale elements independently, which is perfect for performance and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow with no restrictions, begin working with these tools early. They preserve time, cut down threat, and help you remain centered on building, not fixing.
Watch Everything
Should you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your app is undertaking, location problems early, and make far better selections as your application grows. It’s a vital Component of setting up scalable systems.
Commence by tracking standard metrics like CPU use, memory, disk House, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just observe your servers—monitor your app too. Keep an eye on how long it will take for customers to load webpages, how frequently glitches transpire, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or simply a company goes down, you'll want to get notified promptly. This can help you correct concerns quick, frequently before customers even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back before it will cause true harm.
As your application grows, targeted traffic and information maximize. With no monitoring, you’ll miss out on signs of hassle right up until it’s way too late. But with the proper applications in position, you continue to be in control.
To put it briefly, monitoring helps you maintain your application reputable and scalable. It’s not just about spotting failures—it’s about understanding your technique and making sure it really works well, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even small applications have to have a powerful Basis. By designing meticulously, optimizing properly, and utilizing the right equipment, you could Construct applications that grow easily devoid of breaking under pressure. Commence smaller, think huge, and Make smart.